@neodx/log
Powerful lightweight logger for any requirements.
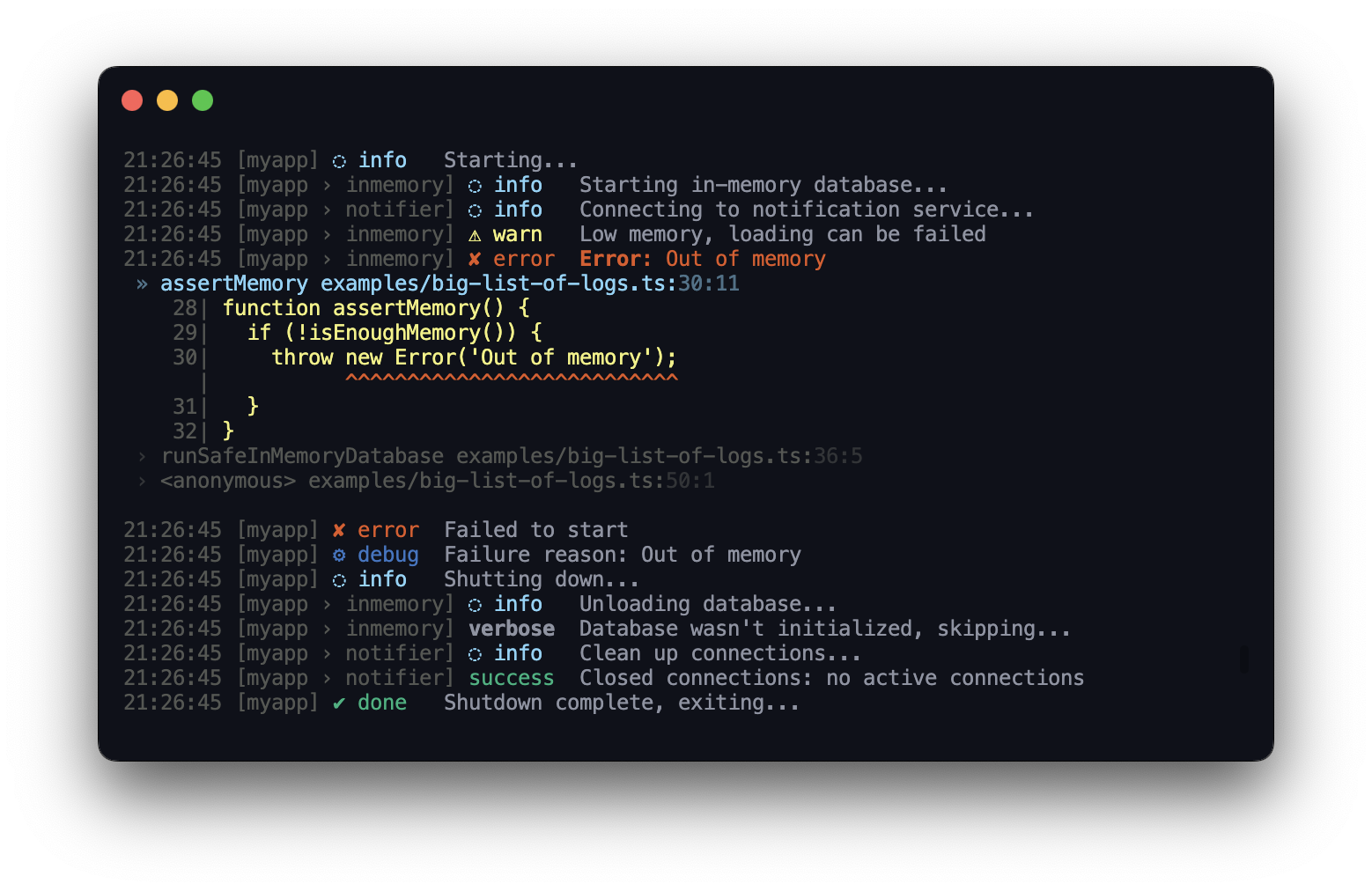
- 🤏 Tiny and simple.
< 1kb!
in browser, no extra configuration - 🚀 Fast enough. No extra overhead, no hidden magic
- 🏗️ Customizable. You can replace core logic and build your own logger from scratch
- 💅 Rich features and DX. JSON logs, pretty dev logs, readable errors, and more
- 👏 Well typed. Written in TypeScript, with full type support
- 🫢 Built-in HTTP frameworks ⛓️ express, koa, Node core
http
loggers are supported out of the box - 👐 Isomorphic. Automatically works in Node.js and browsers
Installation
bash
npm install -D @neodx/log
bash
yarn add -D @neodx/log
bash
pnpm add -D @neodx/log
Getting Started
To begin using @neodx/log
easily, you need to create a logger instance using the createLogger
function.
ts
import { createLogger } from '@neodx/log/node';
const log = createLogger();
log.error(new Error('Something went wrong')); // Something went wrong
log.warn('Be careful!'); // Be careful!
log.info('Hello, world!'); // Hello, world!
log.info({ object: 'property' }, 'Template %s', 'string'); // Template string { object: 'property' }
log.done('Task completed'); // Task completed
log.success('Alias for done');
log.debug('Some additional information...'); // nothing, because debug level is disabled
log.verbose('Alias for debug');
Add child loggers
typescript
const child = log.child('child name');
child.info('message'); // [child name] message
Configure log levels
typescript
const log = createLogger({
level: process.env.NODE_ENV === 'production' ? 'info' : 'debug'
});
log.success('This message will be logged only in development');
See detailed errors in development
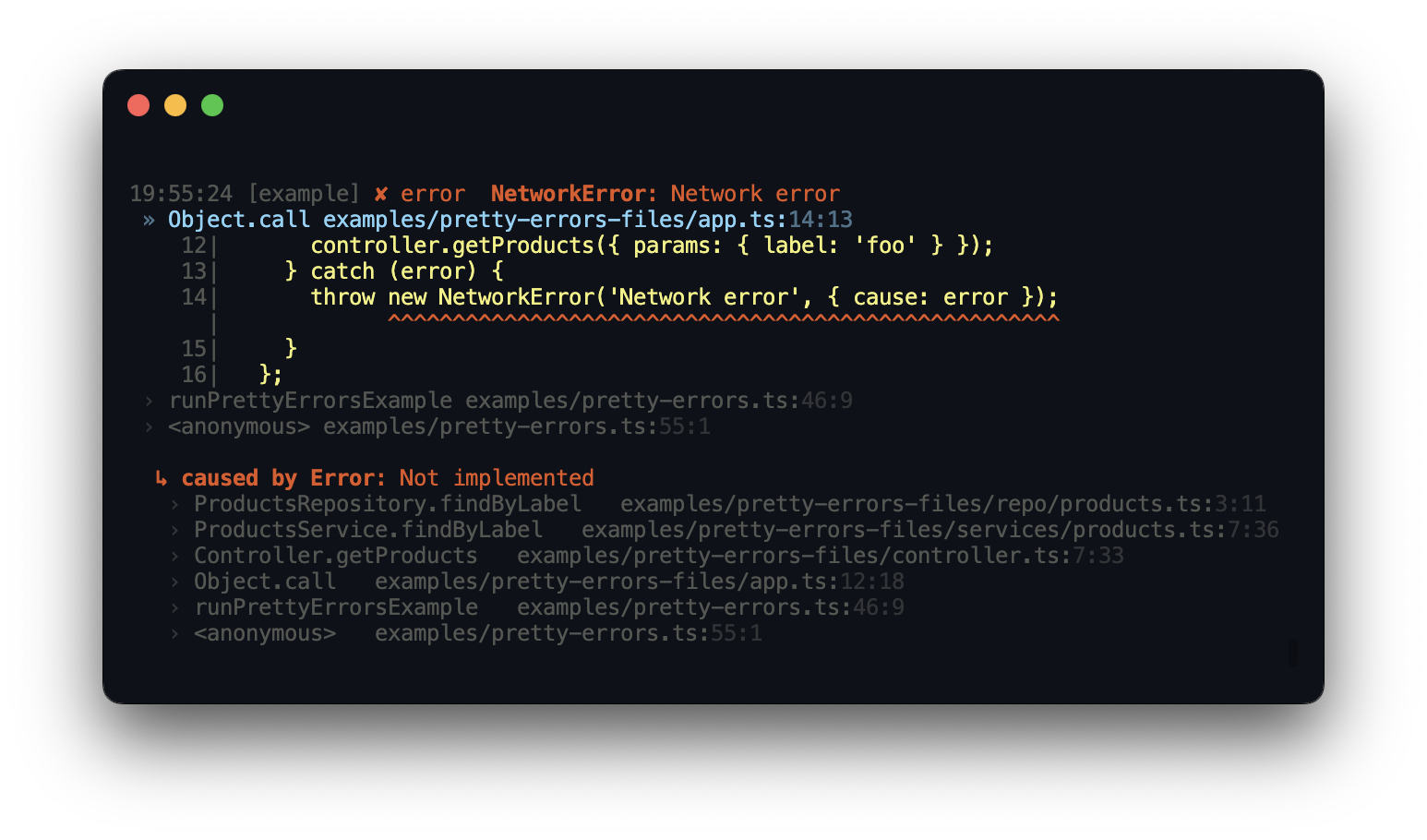
Explore pretty target for details.
Use JSON logs in production
By default,
@neodx/log
already uses pretty logs in development and JSON logs in production for Node.js environment.
typescript
import { createLogger, json, pretty } from '@neodx/log/node';
const log = createLogger({
target: process.env.NODE_ENV === 'production' ? json() : pretty()
});
Integrate with your framework
We're supporting built-in integrations with a some popular HTTP frameworks:
Just add the logger middleware to your app and you're ready to go! For example, for Koa:
typescript
import { createKoaLogger } from '@neodx/log/koa';
// ...
app.use(createKoaLogger());
// ...
function myMiddleware(ctx) {
ctx.log.info('Some log message');
// ...
}